Manage orders with the REST Admin API
You can use the REST Admin API to create orders and transactions to validate your app's behavior.
Tip
Before you begin
To best understand this guide, you can familiarize yourself with the concepts of orders and transactions.
Transactions are associated to orders and occur each time there is an exchange of money. Transactions are processed by payment providers, and involve the transfer of money from the issuer account (the customer's credit card) to the acquirer (the merchant's bank account). For more information, see Getting paid.
Access scopes
To use the Admin API to edit orders, your app needs to request the write_order_edits
access scope for a ShopBase store. For more information on requesting access scopes when your app is installed, see OAuth. To make things easy for you, we have also included a guideline on sending request with Postman.
Create an order
To test orders, create an order with an authorization transaction by sending a POST request to the Order resource:
POST https://shop-name.onshopbase.com/admin/orders.json
{
"order":{
"email":" ",
"financial_status":"pending",
"line_items":[
{
"title":"Big Brown Bear Boots",
"price":100,
"grams":"1300",
"quantity":2
}
]
}
}
SAMPLE RESPOND:
{
"orders":[
{
"id": 5549662,
"currency":"USD",
"customer":{
"accepts_marketing":false,
"average_spent":0,
"default_address":null,
"email":" ",
"first_name":"",
"id":49231780,
"last_name":"",
"last_order_at":0,
"last_order_id":0,
"last_order_name":"",
"note":"",
"orders_count":0,
"phone":"",
"state":"disabled",
"tags":"",
"tax_exempt":false,
"total_spent":0,
"updated_at":"2020-12-15T05:09:48+00:00",
"verified_email":false
},
"customer_locale":"",
"discount_applications":null,
"discount_code":null,
"draft_order_number":0,
"email":" ",
"financial_status":"pending",
"fulfillment_status":"",
...
}
]
}
To be more specific, we can take a look at how we can use Postman to create this order
STEP 1: Create your Shopbase's API Credentials by following the tutorial here.
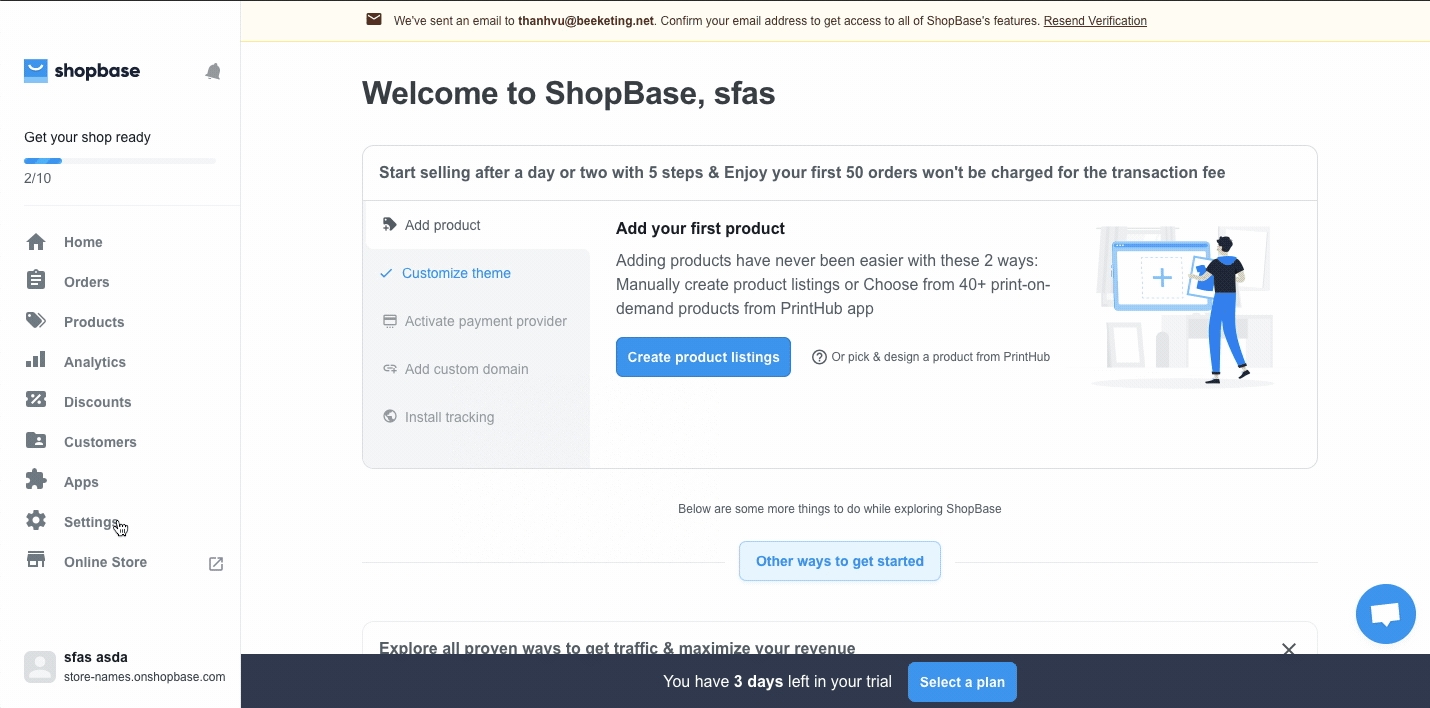
STEP 2: Send the POST Request with Postman

STEP 3: Save this request into a collection and extract the Order ID as a variable

let response = pm.response.json()
pm.globals.set("order_id", response.order.id)

Create the transaction for an order
After creating an order, the returned Order object includes an ID. You can use the order ID to create a transaction.
POST https://shop-name.onshopbase.com/admin/orders/{order_id}/transactions.json
{
"transactions":[
{
"kind":"authorization",
"status":"success",
"amount":200
}
]
}
After you create the transaction, you can then use the order ID to get its transactions and transaction IDs. To retrieve the transactions, send a GET request to the Order Transaction.
GET https://shop-name.onshopbase.com/admin/orders/{order_id}/transactions.json
{
"transactions":[
{
"amount":0,
"application_fee":0,
"authorization":"",
"conversion_rate":0,
"created_at":"2020-12-16T10:45:05+00:00",
"currency":"USD",
"currency_exchange_adjustment":null,
"device_id":0,
"error_code":"",
"gateway":"",
"id":10624938,
"kind":"",
"location_id":0,
"message":"",
"order_id":5557905,
"origin_error_code":"",
"parent_id":0,
"payment_details":null,
"payment_method_id":0,
"processed_at":"",
"receipt":null,
"shop_id":10124799,
"source_name":"",
"staff_id":0,
"status":"",
"test":false,
"transaction_amount":0,
"transaction_currency":"",
"updated_at":"2020-12-16T10:45:05+00:00"
}
]
}
Let's also look at how this request is handled by using Postman:
STEP 1: Use the same API Credential from the above instruction to create a new POST Request. Set `{{order_id}}` parameter in the request url as below:

STEP 2: Save the request call into a collection for future's use.

Last updated
Was this helpful?